インストールされているChromeのヴァージョンに応じて、ChromeDriverを自動で取得・更新してくれる便利なwebdriver_manager。(日頃からSeleniumを使っている人にはお馴染みかと思います。)
今回はChrome Beta版でもwebdriver_managerを使って、ChromeDriverを自動で取得・更新する方法を紹介します。
Chrome Beta版を使用する際でも、手動でドライバーをダウンロードしたり、ヴァージョンを指定したりする手間が無くなります。
コード(Windows・Mac共通)
コードは以下の通りです。
import re
import os
import platform
import requests
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
#コマンドからインストールされているChromeのヴァージョンを取得
def get_chrome_version(cmd):
pattern = r'\d+\.\d+\.\d+'
stdout = os.popen(cmd).read()
version = re.search(pattern, stdout)
chrome_version = version.group(0)
print('Chrome Version : ' + chrome_version)
return chrome_version
#Chromeのヴァージョンから、適合する最新のChromeDriverのヴァージョンを取得
def get_chrome_driver_version(chrome_version):
url = 'https://chromedriver.storage.googleapis.com/LATEST_RELEASE_' + chrome_version
response = requests.request('GET', url)
print('ChromeDriver Version : ' + response.text)
return response.text
#OSを判別
#cmd:それぞれのOSでChrome(Beta)のヴァージョンを確認するコマンド
#location:Chrome(Beta)の場所
pf = platform.system()
if pf == 'Windows':
print('OS : Windows')
cmd = r'reg query "HKEY_CURRENT_USER\Software\Google\Chrome Beta\BLBeacon" /v version'
location = 'C:/Program Files/Google/Chrome Beta/Application/chrome.exe'
elif pf == 'Darwin':
print('OS : Mac')
cmd = r'/Applications/Google\ Chrome\ Beta.app/Contents/MacOS/Google\ Chrome\ Beta --version'
location = '/Applications/Google Chrome Beta.app/Contents/MacOS/Google Chrome Beta'
#Chromeのヴァージョン取得
chrome_version = get_chrome_version(cmd)
#Chromeのヴァージョンから、適合する最新のChromeDriverのヴァージョンを取得
driver_version = get_chrome_driver_version(chrome_version)
#Chromeの場所を指定
options = Options()
options.binary_location = location
#ChromeDriverのヴァージョンを指定
chrome_service = ChromeService(ChromeDriverManager(version=driver_version).install())
driver = webdriver.Chrome(service=chrome_service,options=options)
#google.comを開く
driver.get('https://google.com')
実行するとWindows・ Macそれぞれ以下のような出力がされ、google .comが開かれます。
- ■ Windowsでの出力結果
- OS : Windows
- Chrome Version : 104.0.5112
- ChromeDriver Version : 104.0.5112.20
- ====== WebDriver manager ======
- There is no [win32] chromedriver for browser in cache
- Trying to download new driver from
- https://chromedriver.storage.googleapis.com/104.0.5112.20/chromedriver_win32.zip
- Driver has been saved in cache [C:\Users\micro\.wdm\drivers\chromedriver\win32\104.0.5112.20]
- ■ Macでの出力結果
- OS : Mac
- Chrome Version : 104.0.5112
- ChromeDriver Version : 104.0.5112.20
- ====== WebDriver manager ======
- There is no [mac64] chromedriver for browser in cache
- Trying to download new driver from
- https://chromedriver.storage.googleapis.com/104.0.5112.20/chromedriver_mac64.zip
- Driver has been saved in cache [/Users/webtrair/.wdm/drivers/chromedriver/mac64/104.0.5112.20]
コードの解説
コードの大まかな流れは以下の通りです。
- OSを判別しget_chrome_version('Chromeのヴァージョン確認コマンド')でChrome Beta版のヴァージョンを取得
- 取得したChrome Beta版のヴァージョンから、get_chrome_driver_version('Chromeのヴァージョン')で、そのヴァージョンに適合する最新のChromeDriverのヴァージョンを取得
- webdriver_managerで使用するChromeDriverのヴァージョンを指定
以下では上記のコードを分解して解説しています。
1.Chrome Beta版のヴァージョンを取得
まずOSに応じて、それぞれのコマンドの実行結果から、Chrome Beta版のヴァージョンを取得します。
import re
import os
import platform
#コマンドからインストールされているChromeのヴァージョンを取得
def get_chrome_version(cmd):
pattern = r'\d+\.\d+\.\d+'
stdout = os.popen(cmd).read()
version = re.search(pattern, stdout)
chrome_version = version.group(0)
print('Chrome Version : ' + chrome_version)
return chrome_version
#OSを判別
#cmd:それぞれのOSでChrome(Beta)のヴァージョンを確認するコマンド
#location:Chrome(Beta)の場所
pf = platform.system()
if pf == 'Windows':
print('OS : Windows')
cmd = r'reg query "HKEY_CURRENT_USER\Software\Google\Chrome Beta\BLBeacon" /v version'
location = 'C:/Program Files/Google/Chrome Beta/Application/chrome.exe'
elif pf == 'Darwin':
print('OS : Mac')
cmd = r'/Applications/Google\ Chrome\ Beta.app/Contents/MacOS/Google\ Chrome\ Beta --version'
location = '/Applications/Google Chrome Beta.app/Contents/MacOS/Google Chrome Beta'
chrome_version = get_chrome_version(cmd)
#■出力結果
#>> OS : Windows
#>> Chrome Version : 104.0.5112
2.ChromeDriverのヴァージョンを取得
そもそも「Chromeのヴァージョン ≠ ChromeDriverのヴァージョン」であるから、先に取得したChromeのヴァージョンから、これに適したChromeDriverのヴァージョンを取得する必要がある。(ChromeDriverのヴァージョンが分からないと、version=で指定できないため。)
ここで便利なのが「https://chromedriver.storage.googleapis.com/ LATEST_RELEASE_XXX」
URLのXXXにChromeのヴァージョンを入れてると、そのChromeヴァージョンに適した最新のChromeDriverのヴァージョンを返してくれる。ブラウザで試してみると分かりやすいと思います。
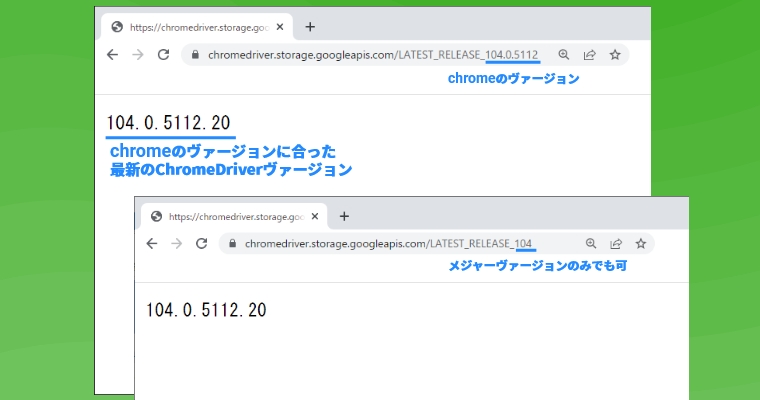
- ■「https://chromedriver.storage.googleapis.com/LATEST_RELEASE_XXX」の使用例
- https://chromedriver.storage.googleapis.com/LATEST_RELEASE_104.0.5112
- > 104.0.5112.20
- メジャー(104)のみでも指定OK
- https://chromedriver.storage.googleapis.com/LATEST_RELEASE_104
- > 104.0.5112.20
- 過去のヴァージョンでも使用可能
- https://chromedriver.storage.googleapis.com/LATEST_RELEASE_72.0.3626
- > 72.0.3626.69
- そもそもLATEST_RELEASEのみだと、現在のStable Release(安定版)の
- 最新のChromeDriverのヴァージョンが返されます。
- https://chromedriver.storage.googleapis.com/LATEST_RELEASE
- > 103.0.5060.53
import requests
def get_chrome_driver_version(chrome_version):
url = 'https://chromedriver.storage.googleapis.com/LATEST_RELEASE_' + chrome_version
response = requests.request('GET', url)
print('ChromeDriver Version : ' + response.text)
return response.text
driver_version = get_chrome_driver_version('104.0.5112')
#■出力結果
#>> ChromeDriver Version : 104.0.5112.20
3.webdriver_managerでChromeDriverのヴァージョンを指定
webdriver_managerでは以下のコードのように「ChromeDriverManager(version='ChromeDriverのヴァージョン')」で、使用するChromeDriverのヴァージョンを指定できる。
from selenium import webdriver
from selenium.webdriver.chrome.options import Options
from selenium.webdriver.chrome.service import Service as ChromeService
from webdriver_manager.chrome import ChromeDriverManager
options = Options()
options.binary_location = 'C:/Program Files/Google/Chrome Beta/Application/chrome.exe'
#以下のように使用するChromeDriverのヴァージョン指定ができる
driver_version = '104.0.5112.20'
chrome_service = ChromeService(ChromeDriverManager(version=driver_version).install())
driver = webdriver.Chrome(service=chrome_service,options=options)
driver.get('https://google.com')
COMMENTS
はじめまして、上のコードを実行すると、以下のエラーが表示されます。
解決方法がわかれば教えてください。
TypeError Traceback (most recent call last)
in
11 chrome_service = ChromeService(ChromeDriverManager(version=driver_version).install())
12
---> 13 driver = webdriver.Chrome(service=chrome_service,options=options)
14
15 driver.get('https://google.com' class="ansi-yellow-intense-fg ansi-bold">)
TypeError: __init__() got an unexpected keyword argument 'service'
コマンドで「pip3 list」を入力し、seleniumのヴァージョンを確認してください。
seleniumが3.X.Xではないでしょうか。
「pip3 install --upgrade selenium」でSeleniumをアップグレードできます。
(本文中に記載してなくて、申し訳ないですが記事のコードはSelenium4用です。Selenium3で動かしたい場合は再度コメントいただければと思います。)
Seleniumをアップグレードしたらうまくいきました。
ありがとうございました。